Authentication API for Swift
Send OTP codes or integrate 2-factor authentication with our API for Swift easily and securely. Try our API for Swift for free. Our team will help you improve the security and authentication of your app on Swift.
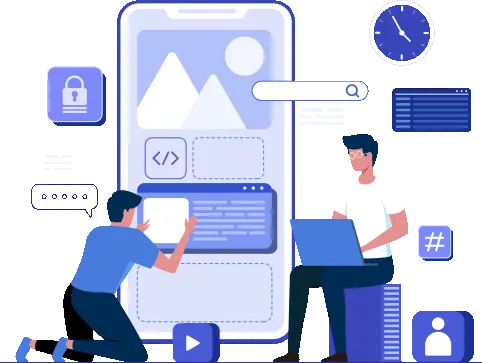
Authentication API functionality
OTP with Swift
let aclass : String = "otp";
let method : String = "generateotp";
let user : String = "user";
let password : String = "password";
let type : String = "number";
let destination : String = "34600000000";
let time : String = "1";
let timetype : String = "days";
let length : String = "6";
let otpformat : String = "all";
let output : String = "";
let urlPath: String = "https://www.afilnet.com/api/http/?class="+aclass+"&method="+method+"&user="+user+"&password="+password+"&type="+type+"&destination="+destination+"&time="+time+"&timetype="+timetype+"&length="+length+"&otpformat="+otpformat+"&output="+output
let url: NSURL = NSURL(string: urlPath)!
let request1: NSURLRequest = NSURLRequest(url: url as URL)
let queue:OperationQueue = OperationQueue()
NSURLConnection.sendAsynchronousRequest(request1 as URLRequest, queue: queue, completionHandler:{ (response: URLResponse?, data: Data?, error: Error?) -> Void in
do {
if let jsonResult = try JSONSerialization.jsonObject(with: data!, options: []) as? NSDictionary {
// Do something with result
}
} catch let error as NSError {
print(error.localizedDescription)
}
})
let aclass : String = "otp";
let method : String = "generateotp";
let user : String = "user";
let password : String = "password";
let type : String = "number";
let destination : String = "34600000000";
let time : String = "1";
let timetype : String = "days";
let length : String = "6";
let otpformat : String = "all";
let output : String = "";
let urlPath: String = "https://www.afilnet.com/api/http/"
let url: NSURL = NSURL(string: urlPath)!
let request1: NSMutableURLRequest = NSMutableURLRequest(url: url as URL)
request1.httpMethod = "POST"
let stringPost="class="+aclass+"&method="+method+"&user="+user+"&password="+password+"&type="+type+"&destination="+destination+"&time="+time+"&timetype="+timetype+"&length="+length+"&otpformat="+otpformat+"&output="+output
let data = stringPost.data(using: String.Encoding.utf8)
request1.timeoutInterval = 60
request1.httpBody=data
request1.httpShouldHandleCookies=false
let queue:OperationQueue = OperationQueue()
NSURLConnection.sendAsynchronousRequest(request1 as URLRequest, queue: queue, completionHandler:{ (response: URLResponse?, data: Data?, error: Error?) -> Void in
do {
if let jsonResult = try JSONSerialization.jsonObject(with: data!, options: []) as? NSDictionary {
// Do something with result
}
} catch let error as Error {
print(error.localizedDescription)
}
Parameter | Description | Compulsory / Optional |
---|---|---|
class=otp | Class requested: Class to which the request is made | Compulsory |
method=generateotp | Class method requested: Method of the class to which the request is made | Compulsory |
user | User and e-mail of your Afilnet account | Compulsory |
password | Password of your Afilnet account | Compulsory |
type | Type of group (email or mobile) | Compulsory |
destination | Mobile number or destination email | Compulsory |
time | Number of days, weeks, months or years | Optional |
timetype | Type of time (Posible value: minutes, hours, days, weeks, months o years) | Optional |
length | Length of the password to generate | Optional |
otpformat | Format of the password to generate, if it includes all the characters, only numbers or only letters (all, numbers, letters) | Optional |
output | Output format of the result | Optional |
Answer:
- status
-
result (if status=success), here you will receive the following values:
- status
- code
- error (if status=error), here you will receive the error code
Error codes:
Code | Description |
---|---|
MISSING_USER | User or email not included |
MISSING_PASSWORD | Password not included |
MISSING_CLASS | Class not included |
MISSING_METHOD | Method not included |
MISSING_COMPULSORY_PARAM | Compulsory parameter not included |
INCORRECT_USER_PASSWORD | Incorrect user or password |
INCORRECT_CLASS | Incorrect class |
INCORRECT_METHOD | Incorrect method |
INCORRECT_TIME_TYPE | Incorrect type of time (possible values: minutes, hours, days, weeks, months or years) |
INCORRECT_TYPE | Incorrect type. The type must be mobile or email |
INCORRECT_FORMAT | The recipient's format is not correct (verify the recipient's email or number) |
INCORRECT_OTP_FORMAT | The OTP format to generate does not exist, this must be one of the following values: all, letters, numbers |
Verify OTP with Swift
let aclass : String = "otp";
let method : String = "generateotp";
let user : String = "user";
let password : String = "password";
let type : String = "number";
let destination : String = "34600000000";
let time : String = "1";
let timetype : String = "days";
let length : String = "6";
let otpformat : String = "all";
let output : String = "";
let urlPath: String = "https://www.afilnet.com/api/http/"
let url: NSURL = NSURL(string: urlPath)!
let request1: NSMutableURLRequest = NSMutableURLRequest(url: url as URL)
request1.httpMethod = "POST"
let stringPost="class="+aclass+"&method="+method+"&user="+user+"&password="+password+"&type="+type+"&destination="+destination+"&time="+time+"&timetype="+timetype+"&length="+length+"&otpformat="+otpformat+"&output="+output
let data = stringPost.data(using: String.Encoding.utf8)
request1.timeoutInterval = 60
request1.httpBody=data
request1.httpShouldHandleCookies=false
let queue:OperationQueue = OperationQueue()
NSURLConnection.sendAsynchronousRequest(request1 as URLRequest, queue: queue, completionHandler:{ (response: URLResponse?, data: Data?, error: Error?) -> Void in
do {
if let jsonResult = try JSONSerialization.jsonObject(with: data!, options: []) as? NSDictionary {
// Do something with result
}
} catch let error as Error {
print(error.localizedDescription)
}let aclass : String = "otp";
let method : String = "verifyotp";
let user : String = "user";
let password : String = "password";
let destination : String = "34600000000";
let code : String = "A73HF3I";
let output : String = "";
let urlPath: String = "https://www.afilnet.com/api/http/?+"&class="+aclass+"&method="+method+"&user="+user+"&password="+password+"&destination="+destination+"&code="+code+"&output="+output
let url: NSURL = NSURL(string: urlPath)!
let request1: NSURLRequest = NSURLRequest(url: url as URL)
let queue:OperationQueue = OperationQueue()
NSURLConnection.sendAsynchronousRequest(request1 as URLRequest, queue: queue, completionHandler:{ (response: URLResponse?, data: Data?, error: Error?) -> Void in
do {
if let jsonResult = try JSONSerialization.jsonObject(with: data!, options: []) as? NSDictionary {
// Do something with result
}
} catch let error as NSError {
print(error.localizedDescription)
}
})
let aclass : String = "otp";
let method : String = "verifyotp";
let user : String = "user";
let password : String = "password";
let destination : String = "34600000000";
let code : String = "A73HF3I";
let output : String = "";
let urlPath: String = "https://www.afilnet.com/api/http/"
let url: NSURL = NSURL(string: urlPath)!
let request1: NSMutableURLRequest = NSMutableURLRequest(url: url as URL)
request1.httpMethod = "POST"
let stringPost="class="+aclass+"&method="+method+"&user="+user+"&password="+password+"&destination="+destination+"&code="+code+"&output="+output
let data = stringPost.data(using: String.Encoding.utf8)
request1.timeoutInterval = 60
request1.httpBody=data
request1.httpShouldHandleCookies=false
let queue:OperationQueue = OperationQueue()
NSURLConnection.sendAsynchronousRequest(request1 as URLRequest, queue: queue, completionHandler:{ (response: URLResponse?, data: Data?, error: Error?) -> Void in
do {
if let jsonResult = try JSONSerialization.jsonObject(with: data!, options: []) as? NSDictionary {
// Do something with result
}
} catch let error as Error {
print(error.localizedDescription)
}
Parameter | Description | Compulsory / Optional |
---|---|---|
class=otp | Class requested: Class to which the request is made | Compulsory |
method=verifyotp | Class method requested: Method of the class to which the request is made | Compulsory |
user | User and e-mail of your Afilnet account | Compulsory |
password | Password of your Afilnet account | Compulsory |
destination | Mobile number or destination email | Compulsory |
code | Password to verify | Optional |
output | Output format of the result | Optional |
Answer:
- status
-
result (if status=success), here you will receive the following values:
- No additional values will be sent to you
- error (if status=error), here you will receive the error code
Error codes:
Code | Description |
---|---|
MISSING_USER | User or email not included |
MISSING_PASSWORD | Password not included |
MISSING_CLASS | Class not included |
MISSING_METHOD | Method not included |
MISSING_COMPULSORY_PARAM | Compulsory parameter not included |
INCORRECT_USER_PASSWORD | Incorrect user or password |
INCORRECT_CLASS | Incorrect class |
INCORRECT_METHOD | Incorrect method |
CODE_NOT_FOUND | The code sent is incorrect or has expired |
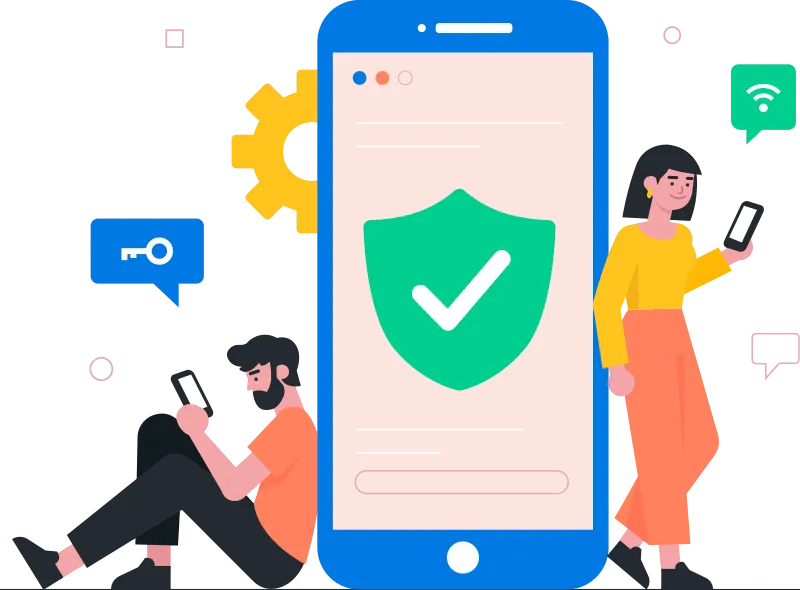
Which API for Swift should I use?
Discover the advantages and disadvantages of each of our APIs. Find out which API is best for your Software in Swift.
This API allows you to connect to us from Swift to send requests via HTTP GET requests. This request sends the parameters in the same URL as the request.
- HTTP GET is extremely simple to implement
- Information is sent unencrypted (passwords could be extracted from logs or cache)
- Maximum request of ~4000 characters
The POST request API allows you to connect to our API from Swift by sending request parameters via HTTP POST parameters. The information is sent independently of the URL.
- HTTP POST is simple to implement
- Information is sent encrypted
- There is no limit on the size of the request
- Medium security
The basic authentication API allows the use of GET and POST requests in Swift with an additional security layer, since in this case the username and password are sent in the header of the request.
- Basic authentication is easy to implement
- Access data is sent encrypted
- The size limit depends on the use of GET or POST
- Medium security
SOAP allows you to send requests in XML format with Swift, SOAP adds an extra layer of security to API requests.
- SOAP integration is more complex
- Information is sent encrypted
- There is no limit on the size of the request
- Medium / High security
Our JSON API allows you to send requests in JSON format with Swift, in addition this API adds the oAuth 2.0 protocol in the authentication that allows you to add an additional layer of security.
- JSON oAuth 2.0 integration is more complex
- Information is sent encrypted
- There is no limit on the size of the request
- High security
Connect Swift with our OTP (One-Time Password) API
Register as a client
In order to have access to the API you must be an Afilnet client. Registration will take a few minutes.
Request your free trial
Our company will offer you trial balance that will allow you to test with the API you need.
Integrate the API
Perform API integration using the programming language of your choice. If you have any questions or suggestions about the API, contact us
Welcome to Afilnet!
Everything ready!, has managed to improve its communications with Afilnet. We are here to support our API when you need it
Contact our team with any questions through the contact methods that we offer. Our team will try to offer you an immediate solution and will help you in the integration of our API in your Software.