Reseller API for Android
Manage your reseller account with our API for Android easily and securely. Try our API for Android for free. Our team will help you manage the clients of your reseller account in Android.
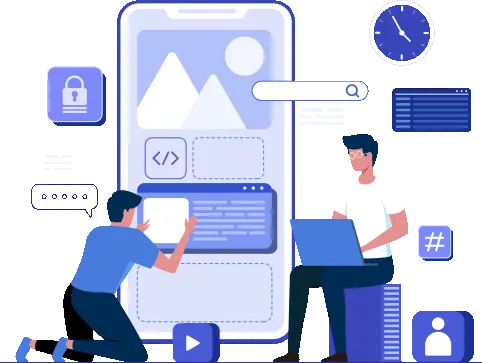
API functionality to Resellers
Add a subaccount with Android
String afilnet_class="subaccount";
String afilnet_method="addsubaccount";
String afilnet_user="user";
String afilnet_password="password";
String afilnet_accountemail="email@email.com";
String afilnet_accountpassword="password";
String afilnet_namelastname="name+lastname";
String afilnet_iscompany="1";
String afilnet_companyname="mycompany";
String afilnet_documentid="123456789A";
String afilnet_language="en";
String afilnet_countrycode="us";
String afilnet_cityid="1";
String afilnet_cityname="town";
String afilnet_zipcode="123456";
String afilnet_address="address";
// Create an URL request
String sUrl = "https://www.afilnet.com/api/http/?class="+afilnet_class+"&method="+afilnet_method+"&user="+afilnet_user+"&password="+afilnet_password+"&accountemail="+afilnet_accountemail+"&accountpassword="+afilnet_accountpassword+"&namelastname="+afilnet_namelastname+"&iscompany="+afilnet_iscompany+"&companyname="+afilnet_companyname+"&documentid="+afilnet_documentid+"&language="+afilnet_language+"&countrycode="+afilnet_countrycode+"&cityid="+afilnet_cityid+"&cityname="+afilnet_cityname+"&zipcode="+afilnet_zipcode+"&address="+afilnet_address;
URL url = new URL(sUrl);
StringBuilder builder = new StringBuilder();
BufferedReader theJSONline = new BufferedReader(new InputStreamReader(url.openStream()));
builder.append(theJSONline.readLine());
String content = builder.toString();
String afilnet_class="subaccount";
String afilnet_method="addsubaccount";
String afilnet_user="user";
String afilnet_password="password";
String afilnet_accountemail="email@email.com";
String afilnet_accountpassword="password";
String afilnet_namelastname="name+lastname";
String afilnet_iscompany="1";
String afilnet_companyname="mycompany";
String afilnet_documentid="123456789A";
String afilnet_language="en";
String afilnet_countrycode="us";
String afilnet_cityid="1";
String afilnet_cityname="town";
String afilnet_zipcode="123456";
String afilnet_address="address";
// Create the POST request
String post = "class="+afilnet_class+"&method="+afilnet_method+"&user="+afilnet_user+"&password="+afilnet_password+"&accountemail="+afilnet_accountemail+"&accountpassword="+afilnet_accountpassword+"&namelastname="+afilnet_namelastname+"&iscompany="+afilnet_iscompany+"&companyname="+afilnet_companyname+"&documentid="+afilnet_documentid+"&language="+afilnet_language+"&countrycode="+afilnet_countrycode+"&cityid="+afilnet_cityid+"&cityname="+afilnet_cityname+"&zipcode="+afilnet_zipcode+"&address="+afilnet_address;
// We generate the URL
URL myurl = new URL("https://www.afilnet.com/api/http/");
// We create the connection
HttpURLConnection con = (HttpURLConnection) myurl.openConnection();
con.setDoOutput(true);
con.setRequestProperty("Content-Type", "application/x-www-form-urlencoded");
con.setRequestProperty("Content-Length", String.valueOf(post.length()));
con.setRequestMethod("POST");
// We build the
OutputStream os = con.getOutputStream();
os.write(post.getBytes());
os.close();
StringBuilder sb = new StringBuilder();
int HttpResult = con.getResponseCode();
if(HttpResult == HttpURLConnection.HTTP_OK)
{
BufferedReader br = new BufferedReader(new InputStreamReader(con.getInputStream(),"utf-8"));
String line = null;
while ((line = br.readLine()) != null) {
sb.append(line + "\n");
}
br.close();
System.out.println(""+sb.toString());
} else {
System.out.println(con.getResponseCode());
System.out.println(con.getResponseMessage());
}
String afilnet_class="subaccount";
String afilnet_method="addsubaccount";
String afilnet_user="user";
String afilnet_password="password";
String afilnet_accountemail="email@email.com";
String afilnet_accountpassword="password";
String afilnet_namelastname="name+lastname";
String afilnet_iscompany="1";
String afilnet_companyname="mycompany";
String afilnet_documentid="123456789A";
String afilnet_language="en";
String afilnet_countrycode="us";
String afilnet_cityid="1";
String afilnet_cityname="town";
String afilnet_zipcode="123456";
String afilnet_address="address";
// Create the POST request
String post = "class="+afilnet_class+"&method="+afilnet_method+"&accountemail="+afilnet_accountemail+"&accountpassword="+afilnet_accountpassword+"&namelastname="+afilnet_namelastname+"&iscompany="+afilnet_iscompany+"&companyname="+afilnet_companyname+"&documentid="+afilnet_documentid+"&language="+afilnet_language+"&countrycode="+afilnet_countrycode+"&cityid="+afilnet_cityid+"&cityname="+afilnet_cityname+"&zipcode="+afilnet_zipcode+"&address="+afilnet_address;
// We generate the URL
URL myurl = new URL("https://www.afilnet.com/api/basic/");
// We create the connection
HttpURLConnection con = (HttpURLConnection) myurl.openConnection();
String encoded = Base64.getEncoder().encodeToString((afilnet_user+":"+afilnet_password).getBytes(StandardCharsets.UTF_8));
con.setRequestProperty("Authorization", "Basic "+encoded);
con.setDoOutput(true);
con.setRequestProperty("Content-Type", "application/x-www-form-urlencoded");
con.setRequestProperty("Content-Length", String.valueOf(post.length()));
con.setRequestMethod("POST");
// We build the
OutputStream os = con.getOutputStream();
os.write(post.getBytes());
os.close();
StringBuilder sb = new StringBuilder();
int HttpResult = con.getResponseCode();
if(HttpResult == HttpURLConnection.HTTP_OK)
{
BufferedReader br = new BufferedReader(new InputStreamReader(con.getInputStream(),"utf-8"));
String line = null;
while ((line = br.readLine()) != null) {
sb.append(line + "\n");
}
br.close();
System.out.println(""+sb.toString());
} else {
System.out.println(con.getResponseCode());
System.out.println(con.getResponseMessage());
}
Parameter | Description | Compulsory / Optional |
---|---|---|
class=subaccount | Class requested: Class to which the request is made | Compulsory |
method=addsubaccount | Class method requested: Method of the class to which the request is made | Compulsory |
user | User and e-mail of your Afilnet account | Compulsory |
password | Password of your Afilnet account | Compulsory |
accountemail | Account email | Compulsory |
accountpassword | Account password | Compulsory |
namelastname | Name and surname of the contact person | Compulsory |
iscompany | (0) If it is a natural person or (1) if it is a company | Compulsory |
companyname | Name of the company (in case it is a company) | Optional |
documentid | Company identification document code | Compulsory |
language | Language of the subaccount. See attached table | Compulsory |
countrycode | ISO country code in ISO-3166-1 ALPHA-2 format | Compulsory |
cityid | City identifier | Compulsory |
cityname | Name of the city or town to which it belongs | Compulsory |
zipcode | Postal Code | Compulsory |
address | Physical address | Compulsory |
Answer:
- status
-
result (if status=success), here you will receive the following values:
- No additional values will be sent to you
- error (if status=error), here you will receive the error code
Error codes:
Code | Description |
---|---|
MISSING_USER | User or email not included |
MISSING_PASSWORD | Password not included |
MISSING_CLASS | Class not included |
MISSING_METHOD | Method not included |
MISSING_COMPULSORY_PARAM | Compulsory parameter not included |
INCORRECT_USER_PASSWORD | Incorrect user or password |
INCORRECT_CLASS | Incorrect class |
INCORRECT_METHOD | Incorrect method |
EMPTY_COUNTRYCODE | The country code is empty |
EMPTY_FIELDS | Some mandatory field is empty |
COUNTRY_NOT_FOUND | There is no country with the indicated code |
EMPTY_CITY | You have not indicated the city |
CITY_NOT_FOUND | The indicated city does not exist |
INCORRECT_EMAIL | The email included is not valid |
INCORRECT_PASSWORD | The password included is not valid |
RESELLER_ACCOUNT_REQUIRED | The user's account does not have the reseller plan active |
Modify a subaccount with Android
String afilnet_class="subaccount";
String afilnet_method="addsubaccount";
String afilnet_user="user";
String afilnet_password="password";
String afilnet_accountemail="email@email.com";
String afilnet_accountpassword="password";
String afilnet_namelastname="name+lastname";
String afilnet_iscompany="1";
String afilnet_companyname="mycompany";
String afilnet_documentid="123456789A";
String afilnet_language="en";
String afilnet_countrycode="us";
String afilnet_cityid="1";
String afilnet_cityname="town";
String afilnet_zipcode="123456";
String afilnet_address="address";
// Create the POST request
String post = "class="+afilnet_class+"&method="+afilnet_method+"&accountemail="+afilnet_accountemail+"&accountpassword="+afilnet_accountpassword+"&namelastname="+afilnet_namelastname+"&iscompany="+afilnet_iscompany+"&companyname="+afilnet_companyname+"&documentid="+afilnet_documentid+"&language="+afilnet_language+"&countrycode="+afilnet_countrycode+"&cityid="+afilnet_cityid+"&cityname="+afilnet_cityname+"&zipcode="+afilnet_zipcode+"&address="+afilnet_address;
// We generate the URL
URL myurl = new URL("https://www.afilnet.com/api/basic/");
// We create the connection
HttpURLConnection con = (HttpURLConnection) myurl.openConnection();
String encoded = Base64.getEncoder().encodeToString((afilnet_user+":"+afilnet_password).getBytes(StandardCharsets.UTF_8));
con.setRequestProperty("Authorization", "Basic "+encoded);
con.setDoOutput(true);
con.setRequestProperty("Content-Type", "application/x-www-form-urlencoded");
con.setRequestProperty("Content-Length", String.valueOf(post.length()));
con.setRequestMethod("POST");
// We build the
OutputStream os = con.getOutputStream();
os.write(post.getBytes());
os.close();
StringBuilder sb = new StringBuilder();
int HttpResult = con.getResponseCode();
if(HttpResult == HttpURLConnection.HTTP_OK)
{
BufferedReader br = new BufferedReader(new InputStreamReader(con.getInputStream(),"utf-8"));
String line = null;
while ((line = br.readLine()) != null) {
sb.append(line + "\n");
}
br.close();
System.out.println(""+sb.toString());
} else {
System.out.println(con.getResponseCode());
System.out.println(con.getResponseMessage());
}String afilnet_class="subaccount";
String afilnet_method="modifysubaccount";
String afilnet_user="user";
String afilnet_password="password";
String afilnet_accountemail="email@email.com";
String afilnet_modifypassword="1";
String afilnet_accountpassword="password";
String afilnet_namelastname="name+lastname";
String afilnet_iscompany="1";
String afilnet_companyname="mycompany";
String afilnet_documentid="123456789A";
String afilnet_language="en";
String afilnet_countrycode="us";
String afilnet_cityid="1";
String afilnet_cityname="town";
String afilnet_zipcode="123456";
String afilnet_address="address";
// Create an URL request
String sUrl = "https://www.afilnet.com/api/http/?class="+afilnet_class+"&method="+afilnet_method+"&user="+afilnet_user+"&password="+afilnet_password+"&accountemail="+afilnet_accountemail+"&modifypassword="+afilnet_modifypassword+"&accountpassword="+afilnet_accountpassword+"&namelastname="+afilnet_namelastname+"&iscompany="+afilnet_iscompany+"&companyname="+afilnet_companyname+"&documentid="+afilnet_documentid+"&language="+afilnet_language+"&countrycode="+afilnet_countrycode+"&cityid="+afilnet_cityid+"&cityname="+afilnet_cityname+"&zipcode="+afilnet_zipcode+"&address="+afilnet_address;
URL url = new URL(sUrl);
StringBuilder builder = new StringBuilder();
BufferedReader theJSONline = new BufferedReader(new InputStreamReader(url.openStream()));
builder.append(theJSONline.readLine());
String content = builder.toString();
String afilnet_class="subaccount";
String afilnet_method="modifysubaccount";
String afilnet_user="user";
String afilnet_password="password";
String afilnet_accountemail="email@email.com";
String afilnet_modifypassword="1";
String afilnet_accountpassword="password";
String afilnet_namelastname="name+lastname";
String afilnet_iscompany="1";
String afilnet_companyname="mycompany";
String afilnet_documentid="123456789A";
String afilnet_language="en";
String afilnet_countrycode="us";
String afilnet_cityid="1";
String afilnet_cityname="town";
String afilnet_zipcode="123456";
String afilnet_address="address";
// Create the POST request
String post = "class="+afilnet_class+"&method="+afilnet_method+"&user="+afilnet_user+"&password="+afilnet_password+"&accountemail="+afilnet_accountemail+"&modifypassword="+afilnet_modifypassword+"&accountpassword="+afilnet_accountpassword+"&namelastname="+afilnet_namelastname+"&iscompany="+afilnet_iscompany+"&companyname="+afilnet_companyname+"&documentid="+afilnet_documentid+"&language="+afilnet_language+"&countrycode="+afilnet_countrycode+"&cityid="+afilnet_cityid+"&cityname="+afilnet_cityname+"&zipcode="+afilnet_zipcode+"&address="+afilnet_address;
// We generate the URL
URL myurl = new URL("https://www.afilnet.com/api/http/");
// We create the connection
HttpURLConnection con = (HttpURLConnection) myurl.openConnection();
con.setDoOutput(true);
con.setRequestProperty("Content-Type", "application/x-www-form-urlencoded");
con.setRequestProperty("Content-Length", String.valueOf(post.length()));
con.setRequestMethod("POST");
// We build the
OutputStream os = con.getOutputStream();
os.write(post.getBytes());
os.close();
StringBuilder sb = new StringBuilder();
int HttpResult = con.getResponseCode();
if(HttpResult == HttpURLConnection.HTTP_OK)
{
BufferedReader br = new BufferedReader(new InputStreamReader(con.getInputStream(),"utf-8"));
String line = null;
while ((line = br.readLine()) != null) {
sb.append(line + "\n");
}
br.close();
System.out.println(""+sb.toString());
} else {
System.out.println(con.getResponseCode());
System.out.println(con.getResponseMessage());
}
String afilnet_class="subaccount";
String afilnet_method="modifysubaccount";
String afilnet_user="user";
String afilnet_password="password";
String afilnet_accountemail="email@email.com";
String afilnet_modifypassword="1";
String afilnet_accountpassword="password";
String afilnet_namelastname="name+lastname";
String afilnet_iscompany="1";
String afilnet_companyname="mycompany";
String afilnet_documentid="123456789A";
String afilnet_language="en";
String afilnet_countrycode="us";
String afilnet_cityid="1";
String afilnet_cityname="town";
String afilnet_zipcode="123456";
String afilnet_address="address";
// Create the POST request
String post = "class="+afilnet_class+"&method="+afilnet_method+"&accountemail="+afilnet_accountemail+"&modifypassword="+afilnet_modifypassword+"&accountpassword="+afilnet_accountpassword+"&namelastname="+afilnet_namelastname+"&iscompany="+afilnet_iscompany+"&companyname="+afilnet_companyname+"&documentid="+afilnet_documentid+"&language="+afilnet_language+"&countrycode="+afilnet_countrycode+"&cityid="+afilnet_cityid+"&cityname="+afilnet_cityname+"&zipcode="+afilnet_zipcode+"&address="+afilnet_address;
// We generate the URL
URL myurl = new URL("https://www.afilnet.com/api/basic/");
// We create the connection
HttpURLConnection con = (HttpURLConnection) myurl.openConnection();
String encoded = Base64.getEncoder().encodeToString((afilnet_user+":"+afilnet_password).getBytes(StandardCharsets.UTF_8));
con.setRequestProperty("Authorization", "Basic "+encoded);
con.setDoOutput(true);
con.setRequestProperty("Content-Type", "application/x-www-form-urlencoded");
con.setRequestProperty("Content-Length", String.valueOf(post.length()));
con.setRequestMethod("POST");
// We build the
OutputStream os = con.getOutputStream();
os.write(post.getBytes());
os.close();
StringBuilder sb = new StringBuilder();
int HttpResult = con.getResponseCode();
if(HttpResult == HttpURLConnection.HTTP_OK)
{
BufferedReader br = new BufferedReader(new InputStreamReader(con.getInputStream(),"utf-8"));
String line = null;
while ((line = br.readLine()) != null) {
sb.append(line + "\n");
}
br.close();
System.out.println(""+sb.toString());
} else {
System.out.println(con.getResponseCode());
System.out.println(con.getResponseMessage());
}
Parameter | Description | Compulsory / Optional |
---|---|---|
class=subaccount | Class requested: Class to which the request is made | Compulsory |
method=modifysubaccount | Class method requested: Method of the class to which the request is made | Compulsory |
user | User and e-mail of your Afilnet account | Compulsory |
password | Password of your Afilnet account | Compulsory |
accountemail | Account email | Compulsory |
modifypassword | Compulsory | |
accountpassword | Account password | Optional |
namelastname | Name and surname of the contact person | Compulsory |
iscompany | (0) If it is a natural person or (1) if it is a company | Compulsory |
companyname | Name of the company (in case it is a company) | Optional |
documentid | Company identification document code | Compulsory |
language | Language of the subaccount. See attached table | Compulsory |
countrycode | ISO country code in ISO-3166-1 ALPHA-2 format | Compulsory |
cityid | City identifier | Compulsory |
cityname | Name of the city or town to which it belongs | Compulsory |
zipcode | Postal Code | Compulsory |
address | Physical address | Compulsory |
Answer:
- status
-
result (if status=success), here you will receive the following values:
- No additional values will be sent to you
- error (if status=error), here you will receive the error code
Error codes:
Code | Description |
---|---|
MISSING_USER | User or email not included |
MISSING_PASSWORD | Password not included |
MISSING_CLASS | Class not included |
MISSING_METHOD | Method not included |
MISSING_COMPULSORY_PARAM | Compulsory parameter not included |
INCORRECT_USER_PASSWORD | Incorrect user or password |
INCORRECT_CLASS | Incorrect class |
INCORRECT_METHOD | Incorrect method |
EMPTY_COUNTRYCODE | The country code is empty |
EMPTY_FIELDS | Some mandatory field is empty |
COUNTRY_NOT_FOUND | There is no country with the indicated code |
EMPTY_CITY | You have not indicated the city |
CITY_NOT_FOUND | The indicated city does not exist |
INCORRECT_EMAIL | The email included is not valid |
INCORRECT_PASSWORD | The password included is not valid |
ACCOUNT_NOT_FOUND | User account has not been found |
Get list of subaccounts with Android
String afilnet_class="subaccount";
String afilnet_method="modifysubaccount";
String afilnet_user="user";
String afilnet_password="password";
String afilnet_accountemail="email@email.com";
String afilnet_modifypassword="1";
String afilnet_accountpassword="password";
String afilnet_namelastname="name+lastname";
String afilnet_iscompany="1";
String afilnet_companyname="mycompany";
String afilnet_documentid="123456789A";
String afilnet_language="en";
String afilnet_countrycode="us";
String afilnet_cityid="1";
String afilnet_cityname="town";
String afilnet_zipcode="123456";
String afilnet_address="address";
// Create the POST request
String post = "class="+afilnet_class+"&method="+afilnet_method+"&accountemail="+afilnet_accountemail+"&modifypassword="+afilnet_modifypassword+"&accountpassword="+afilnet_accountpassword+"&namelastname="+afilnet_namelastname+"&iscompany="+afilnet_iscompany+"&companyname="+afilnet_companyname+"&documentid="+afilnet_documentid+"&language="+afilnet_language+"&countrycode="+afilnet_countrycode+"&cityid="+afilnet_cityid+"&cityname="+afilnet_cityname+"&zipcode="+afilnet_zipcode+"&address="+afilnet_address;
// We generate the URL
URL myurl = new URL("https://www.afilnet.com/api/basic/");
// We create the connection
HttpURLConnection con = (HttpURLConnection) myurl.openConnection();
String encoded = Base64.getEncoder().encodeToString((afilnet_user+":"+afilnet_password).getBytes(StandardCharsets.UTF_8));
con.setRequestProperty("Authorization", "Basic "+encoded);
con.setDoOutput(true);
con.setRequestProperty("Content-Type", "application/x-www-form-urlencoded");
con.setRequestProperty("Content-Length", String.valueOf(post.length()));
con.setRequestMethod("POST");
// We build the
OutputStream os = con.getOutputStream();
os.write(post.getBytes());
os.close();
StringBuilder sb = new StringBuilder();
int HttpResult = con.getResponseCode();
if(HttpResult == HttpURLConnection.HTTP_OK)
{
BufferedReader br = new BufferedReader(new InputStreamReader(con.getInputStream(),"utf-8"));
String line = null;
while ((line = br.readLine()) != null) {
sb.append(line + "\n");
}
br.close();
System.out.println(""+sb.toString());
} else {
System.out.println(con.getResponseCode());
System.out.println(con.getResponseMessage());
}String afilnet_class="subaccount";
String afilnet_method="getsubaccounts";
String afilnet_user="user";
String afilnet_password="password";
// Create an URL request
String sUrl = "https://www.afilnet.com/api/http/?class="+afilnet_class+"&method="+afilnet_method+"&user="+afilnet_user+"&password="+afilnet_password;
URL url = new URL(sUrl);
StringBuilder builder = new StringBuilder();
BufferedReader theJSONline = new BufferedReader(new InputStreamReader(url.openStream()));
builder.append(theJSONline.readLine());
String content = builder.toString();
String afilnet_class="subaccount";
String afilnet_method="getsubaccounts";
String afilnet_user="user";
String afilnet_password="password";
// Create the POST request
String post = "class="+afilnet_class+"&method="+afilnet_method+"&user="+afilnet_user+"&password="+afilnet_password;
// We generate the URL
URL myurl = new URL("https://www.afilnet.com/api/http/");
// We create the connection
HttpURLConnection con = (HttpURLConnection) myurl.openConnection();
con.setDoOutput(true);
con.setRequestProperty("Content-Type", "application/x-www-form-urlencoded");
con.setRequestProperty("Content-Length", String.valueOf(post.length()));
con.setRequestMethod("POST");
// We build the
OutputStream os = con.getOutputStream();
os.write(post.getBytes());
os.close();
StringBuilder sb = new StringBuilder();
int HttpResult = con.getResponseCode();
if(HttpResult == HttpURLConnection.HTTP_OK)
{
BufferedReader br = new BufferedReader(new InputStreamReader(con.getInputStream(),"utf-8"));
String line = null;
while ((line = br.readLine()) != null) {
sb.append(line + "\n");
}
br.close();
System.out.println(""+sb.toString());
} else {
System.out.println(con.getResponseCode());
System.out.println(con.getResponseMessage());
}
String afilnet_class="subaccount";
String afilnet_method="getsubaccounts";
String afilnet_user="user";
String afilnet_password="password";
// Create the POST request
String post = "class="+afilnet_class+"&method="+afilnet_method;
// We generate the URL
URL myurl = new URL("https://www.afilnet.com/api/basic/");
// We create the connection
HttpURLConnection con = (HttpURLConnection) myurl.openConnection();
String encoded = Base64.getEncoder().encodeToString((afilnet_user+":"+afilnet_password).getBytes(StandardCharsets.UTF_8));
con.setRequestProperty("Authorization", "Basic "+encoded);
con.setDoOutput(true);
con.setRequestProperty("Content-Type", "application/x-www-form-urlencoded");
con.setRequestProperty("Content-Length", String.valueOf(post.length()));
con.setRequestMethod("POST");
// We build the
OutputStream os = con.getOutputStream();
os.write(post.getBytes());
os.close();
StringBuilder sb = new StringBuilder();
int HttpResult = con.getResponseCode();
if(HttpResult == HttpURLConnection.HTTP_OK)
{
BufferedReader br = new BufferedReader(new InputStreamReader(con.getInputStream(),"utf-8"));
String line = null;
while ((line = br.readLine()) != null) {
sb.append(line + "\n");
}
br.close();
System.out.println(""+sb.toString());
} else {
System.out.println(con.getResponseCode());
System.out.println(con.getResponseMessage());
}
Parameter | Description | Compulsory / Optional |
---|---|---|
class=subaccount | Class requested: Class to which the request is made | Compulsory |
method=getsubaccounts | Class method requested: Method of the class to which the request is made | Compulsory |
user | User and e-mail of your Afilnet account | Compulsory |
password | Password of your Afilnet account | Compulsory |
Answer:
- status
-
result (if status=success), here you will receive the following values:
- No additional values will be sent to you
- error (if status=error), here you will receive the error code
Error codes:
Code | Description |
---|---|
MISSING_USER | User or email not included |
MISSING_PASSWORD | Password not included |
MISSING_CLASS | Class not included |
MISSING_METHOD | Method not included |
MISSING_COMPULSORY_PARAM | Compulsory parameter not included |
INCORRECT_USER_PASSWORD | Incorrect user or password |
INCORRECT_CLASS | Incorrect class |
INCORRECT_METHOD | Incorrect method |
Delete a subaccount with Android
String afilnet_class="subaccount";
String afilnet_method="getsubaccounts";
String afilnet_user="user";
String afilnet_password="password";
// Create the POST request
String post = "class="+afilnet_class+"&method="+afilnet_method;
// We generate the URL
URL myurl = new URL("https://www.afilnet.com/api/basic/");
// We create the connection
HttpURLConnection con = (HttpURLConnection) myurl.openConnection();
String encoded = Base64.getEncoder().encodeToString((afilnet_user+":"+afilnet_password).getBytes(StandardCharsets.UTF_8));
con.setRequestProperty("Authorization", "Basic "+encoded);
con.setDoOutput(true);
con.setRequestProperty("Content-Type", "application/x-www-form-urlencoded");
con.setRequestProperty("Content-Length", String.valueOf(post.length()));
con.setRequestMethod("POST");
// We build the
OutputStream os = con.getOutputStream();
os.write(post.getBytes());
os.close();
StringBuilder sb = new StringBuilder();
int HttpResult = con.getResponseCode();
if(HttpResult == HttpURLConnection.HTTP_OK)
{
BufferedReader br = new BufferedReader(new InputStreamReader(con.getInputStream(),"utf-8"));
String line = null;
while ((line = br.readLine()) != null) {
sb.append(line + "\n");
}
br.close();
System.out.println(""+sb.toString());
} else {
System.out.println(con.getResponseCode());
System.out.println(con.getResponseMessage());
}String afilnet_class="subaccount";
String afilnet_method="deletesubaccount";
String afilnet_user="user";
String afilnet_password="password";
String afilnet_accountemail="email@email.com";
// Create an URL request
String sUrl = "https://www.afilnet.com/api/http/?class="+afilnet_class+"&method="+afilnet_method+"&user="+afilnet_user+"&password="+afilnet_password+"&accountemail="+afilnet_accountemail;
URL url = new URL(sUrl);
StringBuilder builder = new StringBuilder();
BufferedReader theJSONline = new BufferedReader(new InputStreamReader(url.openStream()));
builder.append(theJSONline.readLine());
String content = builder.toString();
String afilnet_class="subaccount";
String afilnet_method="deletesubaccount";
String afilnet_user="user";
String afilnet_password="password";
String afilnet_accountemail="email@email.com";
// Create the POST request
String post = "class="+afilnet_class+"&method="+afilnet_method+"&user="+afilnet_user+"&password="+afilnet_password+"&accountemail="+afilnet_accountemail;
// We generate the URL
URL myurl = new URL("https://www.afilnet.com/api/http/");
// We create the connection
HttpURLConnection con = (HttpURLConnection) myurl.openConnection();
con.setDoOutput(true);
con.setRequestProperty("Content-Type", "application/x-www-form-urlencoded");
con.setRequestProperty("Content-Length", String.valueOf(post.length()));
con.setRequestMethod("POST");
// We build the
OutputStream os = con.getOutputStream();
os.write(post.getBytes());
os.close();
StringBuilder sb = new StringBuilder();
int HttpResult = con.getResponseCode();
if(HttpResult == HttpURLConnection.HTTP_OK)
{
BufferedReader br = new BufferedReader(new InputStreamReader(con.getInputStream(),"utf-8"));
String line = null;
while ((line = br.readLine()) != null) {
sb.append(line + "\n");
}
br.close();
System.out.println(""+sb.toString());
} else {
System.out.println(con.getResponseCode());
System.out.println(con.getResponseMessage());
}
String afilnet_class="subaccount";
String afilnet_method="deletesubaccount";
String afilnet_user="user";
String afilnet_password="password";
String afilnet_accountemail="email@email.com";
// Create the POST request
String post = "class="+afilnet_class+"&method="+afilnet_method+"&accountemail="+afilnet_accountemail;
// We generate the URL
URL myurl = new URL("https://www.afilnet.com/api/basic/");
// We create the connection
HttpURLConnection con = (HttpURLConnection) myurl.openConnection();
String encoded = Base64.getEncoder().encodeToString((afilnet_user+":"+afilnet_password).getBytes(StandardCharsets.UTF_8));
con.setRequestProperty("Authorization", "Basic "+encoded);
con.setDoOutput(true);
con.setRequestProperty("Content-Type", "application/x-www-form-urlencoded");
con.setRequestProperty("Content-Length", String.valueOf(post.length()));
con.setRequestMethod("POST");
// We build the
OutputStream os = con.getOutputStream();
os.write(post.getBytes());
os.close();
StringBuilder sb = new StringBuilder();
int HttpResult = con.getResponseCode();
if(HttpResult == HttpURLConnection.HTTP_OK)
{
BufferedReader br = new BufferedReader(new InputStreamReader(con.getInputStream(),"utf-8"));
String line = null;
while ((line = br.readLine()) != null) {
sb.append(line + "\n");
}
br.close();
System.out.println(""+sb.toString());
} else {
System.out.println(con.getResponseCode());
System.out.println(con.getResponseMessage());
}
Parameter | Description | Compulsory / Optional |
---|---|---|
class=subaccount | Class requested: Class to which the request is made | Compulsory |
method=deletesubaccount | Class method requested: Method of the class to which the request is made | Compulsory |
user | User and e-mail of your Afilnet account | Compulsory |
password | Password of your Afilnet account | Compulsory |
accountemail | Account email | Compulsory |
Answer:
- status
-
result (if status=success), here you will receive the following values:
- No additional values will be sent to you
- error (if status=error), here you will receive the error code
Error codes:
Code | Description |
---|---|
MISSING_USER | User or email not included |
MISSING_PASSWORD | Password not included |
MISSING_CLASS | Class not included |
MISSING_METHOD | Method not included |
MISSING_COMPULSORY_PARAM | Compulsory parameter not included |
INCORRECT_USER_PASSWORD | Incorrect user or password |
INCORRECT_CLASS | Incorrect class |
INCORRECT_METHOD | Incorrect method |
ACCOUNT_NOT_FOUND | User account has not been found |
Transfer balance to a subaccount with Android
String afilnet_class="subaccount";
String afilnet_method="deletesubaccount";
String afilnet_user="user";
String afilnet_password="password";
String afilnet_accountemail="email@email.com";
// Create the POST request
String post = "class="+afilnet_class+"&method="+afilnet_method+"&accountemail="+afilnet_accountemail;
// We generate the URL
URL myurl = new URL("https://www.afilnet.com/api/basic/");
// We create the connection
HttpURLConnection con = (HttpURLConnection) myurl.openConnection();
String encoded = Base64.getEncoder().encodeToString((afilnet_user+":"+afilnet_password).getBytes(StandardCharsets.UTF_8));
con.setRequestProperty("Authorization", "Basic "+encoded);
con.setDoOutput(true);
con.setRequestProperty("Content-Type", "application/x-www-form-urlencoded");
con.setRequestProperty("Content-Length", String.valueOf(post.length()));
con.setRequestMethod("POST");
// We build the
OutputStream os = con.getOutputStream();
os.write(post.getBytes());
os.close();
StringBuilder sb = new StringBuilder();
int HttpResult = con.getResponseCode();
if(HttpResult == HttpURLConnection.HTTP_OK)
{
BufferedReader br = new BufferedReader(new InputStreamReader(con.getInputStream(),"utf-8"));
String line = null;
while ((line = br.readLine()) != null) {
sb.append(line + "\n");
}
br.close();
System.out.println(""+sb.toString());
} else {
System.out.println(con.getResponseCode());
System.out.println(con.getResponseMessage());
}String afilnet_class="subaccount";
String afilnet_method="transferbalance";
String afilnet_user="user";
String afilnet_password="password";
String afilnet_accountemail="email@email.com";
String afilnet_balancetotransfer="10";
// Create an URL request
String sUrl = "https://www.afilnet.com/api/http/?class="+afilnet_class+"&method="+afilnet_method+"&user="+afilnet_user+"&password="+afilnet_password+"&accountemail="+afilnet_accountemail+"&balancetotransfer="+afilnet_balancetotransfer;
URL url = new URL(sUrl);
StringBuilder builder = new StringBuilder();
BufferedReader theJSONline = new BufferedReader(new InputStreamReader(url.openStream()));
builder.append(theJSONline.readLine());
String content = builder.toString();
String afilnet_class="subaccount";
String afilnet_method="transferbalance";
String afilnet_user="user";
String afilnet_password="password";
String afilnet_accountemail="email@email.com";
String afilnet_balancetotransfer="10";
// Create the POST request
String post = "class="+afilnet_class+"&method="+afilnet_method+"&user="+afilnet_user+"&password="+afilnet_password+"&accountemail="+afilnet_accountemail+"&balancetotransfer="+afilnet_balancetotransfer;
// We generate the URL
URL myurl = new URL("https://www.afilnet.com/api/http/");
// We create the connection
HttpURLConnection con = (HttpURLConnection) myurl.openConnection();
con.setDoOutput(true);
con.setRequestProperty("Content-Type", "application/x-www-form-urlencoded");
con.setRequestProperty("Content-Length", String.valueOf(post.length()));
con.setRequestMethod("POST");
// We build the
OutputStream os = con.getOutputStream();
os.write(post.getBytes());
os.close();
StringBuilder sb = new StringBuilder();
int HttpResult = con.getResponseCode();
if(HttpResult == HttpURLConnection.HTTP_OK)
{
BufferedReader br = new BufferedReader(new InputStreamReader(con.getInputStream(),"utf-8"));
String line = null;
while ((line = br.readLine()) != null) {
sb.append(line + "\n");
}
br.close();
System.out.println(""+sb.toString());
} else {
System.out.println(con.getResponseCode());
System.out.println(con.getResponseMessage());
}
String afilnet_class="subaccount";
String afilnet_method="transferbalance";
String afilnet_user="user";
String afilnet_password="password";
String afilnet_accountemail="email@email.com";
String afilnet_balancetotransfer="10";
// Create the POST request
String post = "class="+afilnet_class+"&method="+afilnet_method+"&accountemail="+afilnet_accountemail+"&balancetotransfer="+afilnet_balancetotransfer;
// We generate the URL
URL myurl = new URL("https://www.afilnet.com/api/basic/");
// We create the connection
HttpURLConnection con = (HttpURLConnection) myurl.openConnection();
String encoded = Base64.getEncoder().encodeToString((afilnet_user+":"+afilnet_password).getBytes(StandardCharsets.UTF_8));
con.setRequestProperty("Authorization", "Basic "+encoded);
con.setDoOutput(true);
con.setRequestProperty("Content-Type", "application/x-www-form-urlencoded");
con.setRequestProperty("Content-Length", String.valueOf(post.length()));
con.setRequestMethod("POST");
// We build the
OutputStream os = con.getOutputStream();
os.write(post.getBytes());
os.close();
StringBuilder sb = new StringBuilder();
int HttpResult = con.getResponseCode();
if(HttpResult == HttpURLConnection.HTTP_OK)
{
BufferedReader br = new BufferedReader(new InputStreamReader(con.getInputStream(),"utf-8"));
String line = null;
while ((line = br.readLine()) != null) {
sb.append(line + "\n");
}
br.close();
System.out.println(""+sb.toString());
} else {
System.out.println(con.getResponseCode());
System.out.println(con.getResponseMessage());
}
Parameter | Description | Compulsory / Optional |
---|---|---|
class=subaccount | Class requested: Class to which the request is made | Compulsory |
method=transferbalance | Class method requested: Method of the class to which the request is made | Compulsory |
user | User and e-mail of your Afilnet account | Compulsory |
password | Password of your Afilnet account | Compulsory |
accountemail | Account email | Compulsory |
balancetotransfer | Indicates the balance to be transferred to the subaccount, a negative value can be used to subtract the balance from the subaccount. | Compulsory |
Answer:
- status
-
result (if status=success), here you will receive the following values:
- No additional values will be sent to you
- error (if status=error), here you will receive the error code
Error codes:
Code | Description |
---|---|
MISSING_USER | User or email not included |
MISSING_PASSWORD | Password not included |
MISSING_CLASS | Class not included |
MISSING_METHOD | Method not included |
MISSING_COMPULSORY_PARAM | Compulsory parameter not included |
INCORRECT_USER_PASSWORD | Incorrect user or password |
INCORRECT_CLASS | Incorrect class |
INCORRECT_METHOD | Incorrect method |
ACCOUNT_NOT_FOUND | User account has not been found |
NOT_ENOUGH_BALANCE | ERROR_NOT_ENOUGH_BALANCE |
INCORRECT_MIN_BALANCE | ERROR_INCORRECT_MIN_BALANCE |
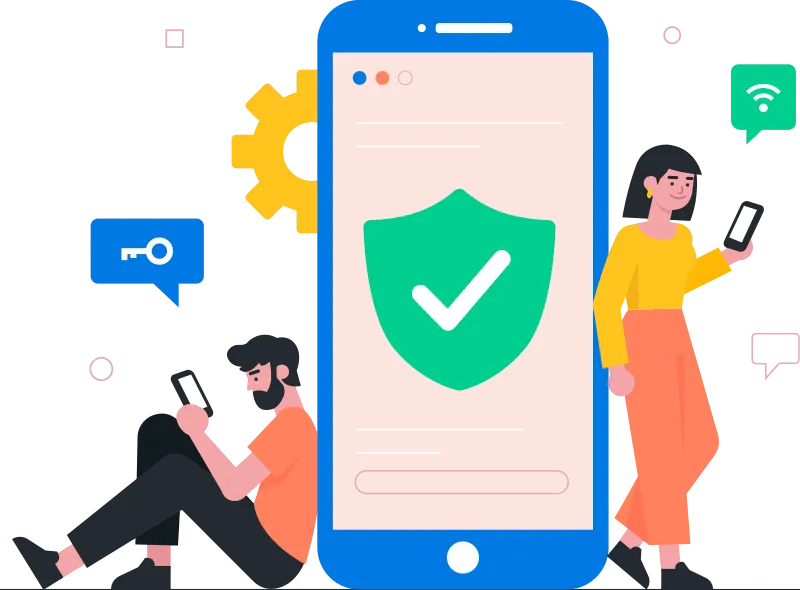
Which API for Android should I use?
Discover the advantages and disadvantages of each of our APIs. Find out which API is best for your Software in Android.
This API allows you to connect to us from Android to send requests via HTTP GET requests. This request sends the parameters in the same URL as the request.
- HTTP GET is extremely simple to implement
- Information is sent unencrypted (passwords could be extracted from logs or cache)
- Maximum request of ~4000 characters
The POST request API allows you to connect to our API from Android by sending request parameters via HTTP POST parameters. The information is sent independently of the URL.
- HTTP POST is simple to implement
- Information is sent encrypted
- There is no limit on the size of the request
- Medium security
The basic authentication API allows the use of GET and POST requests in Android with an additional security layer, since in this case the username and password are sent in the header of the request.
- Basic authentication is easy to implement
- Access data is sent encrypted
- The size limit depends on the use of GET or POST
- Medium security
SOAP allows you to send requests in XML format with Android, SOAP adds an extra layer of security to API requests.
- SOAP integration is more complex
- Information is sent encrypted
- There is no limit on the size of the request
- Medium / High security
Our JSON API allows you to send requests in JSON format with Android, in addition this API adds the oAuth 2.0 protocol in the authentication that allows you to add an additional layer of security.
- JSON oAuth 2.0 integration is more complex
- Information is sent encrypted
- There is no limit on the size of the request
- High security
Connect Android with our Subaccounts API
Register as a client
In order to have access to the API you must be an Afilnet client. Registration will take a few minutes.
Request your free trial
Our company will offer you trial balance that will allow you to test with the API you need.
Integrate the API
Perform API integration using the programming language of your choice. If you have any questions or suggestions about the API, contact us
Welcome to Afilnet!
Everything ready!, has managed to improve its communications with Afilnet. We are here to support our API when you need it
Contact our team with any questions through the contact methods that we offer. Our team will try to offer you an immediate solution and will help you in the integration of our API in your Software.